UnityでVampire survivers 風のゲームを作ろう!#0【連載目次】
レベルが上がった時の特典を実装していきます。
まずは今回やろうとしていることの流れを説明します。
レベルが上がった時にアイテムが表示されるパネルを作ります。
画像が三つ表示されていて、その画像のオブジェクトにEventTriggerをアタッチすることで押されたアイテムが追加される仕組みをもたせます。
クリックされたことを認識するスクリプトを読み込ませます。
そしてクリックされたアイテムを生成することにします。
アイテム表示の為のパネルの構成要素
LevelupUI>Panel>ItemImage1,2,3
はじめに、Create>UI>Canvasを作成、名前をLevelUpUIにします。
LayerをUIに設定します。LevelUpUIをもともとあったCanvasの子要素にします。

LevelUpUIの子要素としてUI>Panelを作成します。
RectTransformLeft 50Right -1000Top 50Bottom -1800
colorは4653DBにしますAlpha値は255にしますAddComoponentでShadowを追加し、X10 Y-10にします。ScaleをXYともに0.7にします。
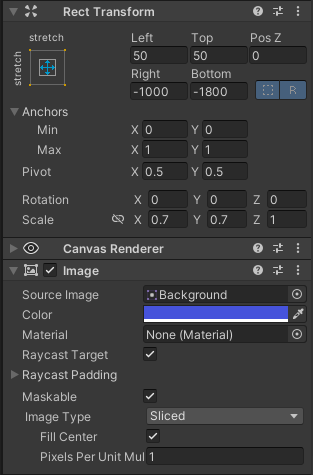
VerticalLayoutGroupをAddComoponentから追加してください。
このComponent持つオブジェクトの中に、垂直に子要素を並べることができます。
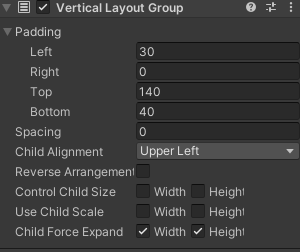
並び方を整えるために、PanelのVerticalLayoutGroupのPaddingを変えていきます。
paddingというのは余白のことです。
Left 30
Right 0
Top 140
Bottom 40
にします。
子要素としてCreate>UI>Imageで3つ並べます。
それぞれitempanel1,itempanel2,itempanel3と名付けます。
Imageに対応した画像を読み込ませていきます。
itempanel
width 900
Height 400
Outline
X10 Y -10
これでレベルが上がった時に表示するためのパネルができました。
このUIも普段は非表示にしておきたいので、LevelUpUIのcanvasのチェックマークを外しておきます。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class ExpManeger : MonoBehaviour
{
public static ExpManeger instance;
[SerializeField] GameObject LevelUPpanelUI;
[SerializeField] Text LevelText;
[SerializeField] Text ItemName;
[SerializeField] Transform PlayerTrans;
[SerializeField] GameObject Particle;
[SerializeField] Text LevelUPText;
public GamemanegerScript GMscript;
public static int currentExp;
public static int CurrentLv;
int NextLevel;
int NeedEXP;
int CumEXP;//累計経験値
public int[,] EXP =//レベルと必要経験値
{ { 1, 0},
{2, 6},
{3, 8},
{4, 10},
{5, 14},
{6, 18},
{7, 20},
{8, 25},
{9, 30} ,
{10, 35},
{11, 40},
{12, 50},
{13, 60},
{14, 70},
{15,5000},
};
Vector2 PlayerPos;
public Slider EXPBar;
public AudioClip sound;
AudioSource audioSource;
// Start is called before the first frame update
void Start() {
currentExp=0;
CurrentLv=1;
NextLevel = CurrentLv + 1;
NeedEXP =[CurrentLv,1];
if (instance == null)
{
instance = this;
}
if (EXPBar != null)
{
EXPBar.maxValue= NeedEXP;
EXPBar.value = currentExp;
}
audioSource = GetComponent<AudioSource>();
}
// Update is called once per frame
void Update()
{//今の経験値と必要経験値を比べて今の経験値が上だったらレベルをあげる
if (currentExp >= EXP[CurrentLv, 1]) {
Debug.Log("レベルアップ"+CurrentLv +"→"+ NextLevel);
CurrentLv += 1;
NextLevel += 1;
LevelText.text = "Level:"+CurrentLv.ToString();
StartCoroutine(LevelUP());
}
}
private IEnumerator LevelUP()
{
audioSource.PlayOneShot(sound);
PlayerPos = Player.transform.position;
currentExp = 0;//現在の経験値を0にする
EXPBar.maxValue= NeedEXP;
EXPBar.value = currentExp;
var confetti = Instantiate(Particle, PlayerPos, transform.rotation);
leveluppanel();
LevelUPText.GetComponent<Text>().enabled = true;
LevelUPpanelUI.GetComponent<Canvas>().enabled = true;
yield return new WaitForSeconds(2);//コルーチンで2秒遅延
LevelUPText.GetComponent<Text>().enabled = false;
}
public void leveluppanel() {
LevelUPpanelUI.GetComponent<Canvas>().enabled = true;
Time.timeScale = 0;//ポーズをする
}
public void ExpBarDraw() {//経験値を拾った時にEXPorbから呼び出される
CumEXP++;
currentExp++;
EXPBar.value = currentExp;
}
}
}
レベルが上がった時にパネルを表示するようにします。EXPManegerのLevelUPpanelUIにLevelupUIをアサインします。次にitempanelに読み込ませるScriptを書いていきます。
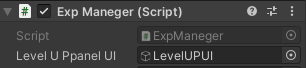
次にitempanelに読み込ませるScriptを書いていきます。パネルの中からどのアイテムが選ばれたかによって処理を変えます。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class LevelupPanel : MonoBehaviour
{
public static LevelupPanel instance;
Text itemText;
public GamemanegerScript GMscript;
string ItemName;
[SerializeField] GameObject LevelUPUI;
Image itemimage;
[SerializeField] Sprite imageFunnel;
[SerializeField] Sprite imageTsuba;
public int argmentrnd;
public Image Imagename;
//public GamemanegerScript GMscript;
[SerializeField] GameObject drone;
[SerializeField] StatusData statusdata;
// Start is called before the first frame update
void Start()
{
if (instance == null)
{
instance = this;
}
}
// Update is called once per frame
void Update()
{
}
public void LevelPanelprocess()
{
//argmentrnd = Random.Range(0, 2);
//itemimage = this.GetComponentInChildren<Image>();
//if (argmentrnd == 0)
//{
// Debug.Log(argmentrnd);
// itemimage.sprite = imageFunnel;
// GMscript.gameObject.GetComponent<GamemanegerScript>().ItemNum(argmentrnd);
//}
//if (argmentrnd == 1)
//{
// Debug.Log(argmentrnd);
// itemimage.sprite = imageTsuba;
// GMscript.gameObject.GetComponent<GamemanegerScript>().ItemNum(argmentrnd);
//}
}
public void Onclick()
{
Imagename=this.gameObject.GetComponent<Image>();
Debug.Log(Imagename.sprite.name);
if (Imagename.sprite.name == "ItemPanel0") {
var Drone = Instantiate(drone, transform.position, transform.rotation);//ドローンの生成
}
if (Imagename.sprite.name == "ItemPanel1") {
Debug.Log("パンチを選択");
statusdata.ATK++;
}
if (Imagename.sprite.name == "ItemPanel2") {
Debug.Log("聖水を選択");
}
Time.timeScale = 1;
LevelUPUI.GetComponent<Canvas>().enabled = false;
}
}
Add Component でEvent Trriger を追加します。
PointerClickを選択し、itempanel0をアサインします。
LevelupPanel.Onclickを選択します。
クリックされたときに自分の持つスプライトの名前を参照することでそれに対応した処理を行うようにしています。
ItemPanel0がクリックされたときにはドローンを生成します。
ItemPanel1の場合は攻撃力を1上昇させます。
ItemPanel2がクリックされた場合の処理は次回以降実装していきます。
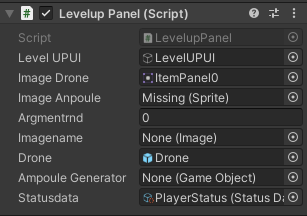
コメント