UnityでVampire survivers 風のゲームを作ろう!#0【連載目次】
ボスの生成
今回はボスの実装をやっていきます。
Enemy1を複製し、名前をBossに変えます。
ScaleをX2 Y2 Z1にします。
ColorをBC00FFにします。
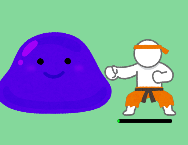
ScriptsフォルダのStatusDataを編集します。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
[CreateAssetMenu(menuName = "Data/Create StatusData")]
public class StatusData : ScriptableObject
{
public string NAME; //キャラ名
public float MAXHP; //最大HP
public float ATK; //攻撃力
public int DEF; //防御力
public float SPEED; //移動速度
public float NockBack; //のけぞり
public float SPAN; //間隔
public int EXP; //経験値
public bool Boss;//ボスか雑魚敵か
}
ScriptableObjectフォルダのenemy1を複製し名前をBossStatusにします。
MaxHp200
ATK1
DEF 3
SPEED 0.6
Nock Back 0
SPAN 0.1
EXP 0
Boss☑
にします。
BossにBossStatusをアサインします。
EnemyGeneratorScriptを変更していきます。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EnemyGeneratorScript : MonoBehaviour
{
[SerializeField]
private GameObject EnemyPrefab;//生成する用の敵キャラPrefabを読み込む
[SerializeField]
private GameObject enemychestPrefab;
[SerializeField]
private GameObject EliteenemyPrefab;
[SerializeField]
private GameObject BossPrefab;
GameObject Player;
Vector2 PlayerPos;//キャラクターの位置を代入する変数
private float currentTime = 0f;
private float span =3f;
//生成される方向を決める乱数用の変数
int rndUD;(上下)
int rndLR;//(左右)
Vector2 enemyspwnPos;//生成される位置
bool Chest1;
bool Chest2;
bool Boss;
void Start()
{
Player = GameObject.FindGameObjectWithTag("Player");//Playerというタグを検索し、見つかったオブジェクトを代入する
}
void Update(){
currentTime += Time.deltaTime;//時間経過をcurrentTimeに代入し時間を測る
if (Time.time<90f) {
if (currentTime > span)//spanで設定した3秒を越えたら処理を実行
{
EnemyGenerate(EnemyPrefab);
currentTime = 0f;
}
if (Time.time > 20&&Chest1== false){
Chest1=true;
EnemyGenerate(enemychestPrefab);
currentTime = 0f;
}
}
if (90f < Time.time&& Time.time < 150f){
if (currentTime > span)//3秒ごと
{
// Debug.Log(span);
EnemyGenerate(ElitenemyPrefab);
currentTime = 0f;
}
if (Time.time > 120f && Chest2 == false)
{
Chest2 = true;
EnemyGenerate(enemychestPrefab);
currentTime = 0f;
}
if (150f < Time.time && Boss == false)
{
Boss = true;
EnemyGenerate(BossPrefab);
}
}
}
~省略~
Clearした演出用のTextを実装していきます。
CanvasにCreate UI>Legacy>Textを作成し、名前をGameClearUIにします。
Colorはff0000にします。
RectTransformにWidth 1080 Height 600にします。
TextにGameClear!と入力し、FontSizeを180にします。
フォントを真ん中揃えにします。
Textのチェックマークを外します。
AddComponentでOutlineを追加します。
X8 Y8にします。
Colorはffffffにします。
続いて、EnemyScriptを変更していきます。
・ボスの生成
・ボスを倒した時の演出
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class EnemyScript : MonoBehaviour
{
GameObject Player;
Vector3 PlayerPos;
[SerializeField] StatusData statusdata;
public bool once = true;
[SerializeField] GameObject punchefect;
Vector3 punchpos;☑
int HP;
bool MUTEKI;//攻撃を受けるかどうかの切り替えを行う
private float currentTime = 0f;
float LifetimeCount = 0f;
Vector3 diff;//プレイヤーとの距離を測るための
Vector3 vector;
private Rigidbody2D rb;
public bool clearbool;
[SerializeField]
GameObject confetti;
[SerializeField]
GameObject GameClear;
void Update(){
~省略~
if (MUTEKI)//攻撃を受けてから0.2秒後にする処理
{
currentTime += Time.deltaTime;
if (currentTime > statusdata.SPAN )
{
currentTime = 0f;
MUTEKI = false;//無敵状態終わらせる
rb.velocity = new Vector2(0, 0);//ノックバックをとめる
punchefect.GetComponent<SpriteRenderer>().enabled = false; //ヒットマーク画像を非表示に戻す
}
}
if (statusdata.Boss == true && clearbool == false)//ボスを倒した時一回だけ処理させる
{
clearbool = true;
GameClear = GameObject.Find("GameClearUI");
GameClear.GetComponent<Text>().enabled = true;
var confe = Instantiate(confetti, this.transform.position, transform.rotation);
StartCoroutine("GameClearfunc");
for (int i=0;i<100;i++) {
Instantiate(confetti, this.transform.position, transform.rotation);
}
Debug.Log("ゲームクリア");
}
if (HP <= 0)//HPが0以下になったら消える
{
punchpos = this.transform.position;
punchpos.z = -2f;
punchefect.transform.position = punchpos;
punchefect.GetComponent<SpriteRenderer>().enabled = true;
LifetimeCount += Time.deltaTime;
if (LifetimeCount > Lifetime)
{
for (int i=0; statusdata.EXP > i; i++) {
var zerachin = Instantiate(EXP_prefab, transform.position, transform.rotation);
if(ChestPrefab!=null){
var Chest = Instantiate(ChestPrefab, transform.position, transform.rotation);}
}
Destroy(this.gameObject);
}
IEnumerator GameClearfunc()
{
GetComponent<SpriteRenderer>().enabled = false;
GetComponent<BoxCollider2D>().enabled = false;
Hitmark.GetComponent<SpriteRenderer>().enabled = false;
yield return new WaitForSeconds(1f);
}
}
~省略~
public bool clearbool;
[SerializeField] GameObject confetti;
[SerializeField] GameObject GameClear;
Clearboolで一度だけ処理をするようにしています。
1度に100個経験値オーブを発生させてクリアした時のお祭り感を出します。
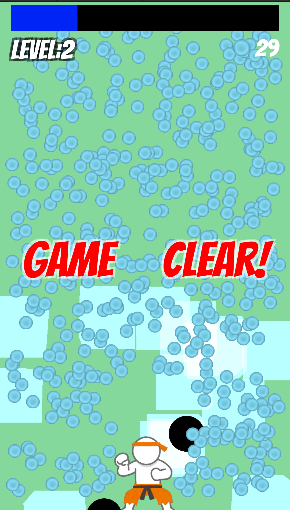
EXPorbのPrefabを複製し、EXPParticleを作ります。
EXPOrbScriptの代わりに使うScriptを作成します。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EXPparticle : MonoBehaviour
{
Vector2 DestinationPos;
Vector2 currentPos;
Vector3 localAngle;
Transform myTransform;
// Start is called before the first frame update
void Start()
{
float rndPosX = Random.Range(-2.6f, 2.61f);//画面の横の長さ
float rndPosY = Random.Range(-4.5f, 4.51f);//画面の縦の長さ
currentPos.x = rndPosX;//ランダム値を代入する
currentPos.y = 6f;//Y軸を表示範囲よりも上に設定しておく
transform.position = currentPos;//現在位置を変える
DestinationPos = transform.position;//目的値のX軸を代入
DestinationPos.y = rndPosY;//目的値のY軸を代入
}
// Update is called once per frame
void Update()
{
transform.position = Vector2.MoveTowards(transform.position, DestinationPos, 4 * Time.deltaTime);
currentPos = transform.position;
if (currentPos.y == DestinationPos.y)
{
}
}
private void OnTriggerEnter2D(Collider2D other)
{
if (other.gameObject.tag == "Player")
{
ExpManeger.instance.ExpBarDraw();
Destroy(this.gameObject);
}
}
}
ampouleのScriptを流用して上から降ってくるようにしました。
EXPorbScriptを削除して変わりにExpParticleを追加しましょう。
GameClearにはGameClearUIをアサインします。
confettiにはExpParticleをアサインします。これでボスを倒した後の演出が実装できました。BossのHPを下げて倒しやすくした状態でヒエラルキー上にドラッグ&ドロップしてみて想定通りの処理が行われているか確認しましょう。
コメント